Introduction to NumPy: A Comprehensive Guide for Beginners

NumPy, short for Numerical Python, is a powerful library in Python specifically designed for working with multi-dimensional arrays. Created in 2005 by merging Numarray into Numeric, NumPy has since become an essential tool for scientific computing in Python. It serves as a foundation for various other scientific libraries such as SciPy, Pandas, and Scikit-learn.
Key Features of NumPy:
- Efficient Data Structures: NumPy arrays, also known as ndarrays, store elements of the same type and size, making them highly efficient for data manipulation.
- High Performance: NumPy offers efficient storage and operations on arrays, even as they grow in size, making it ideal for numerical computations.
- Python Interface: NumPy provides a convenient Python interface for working with multi-dimensional arrays, making it easy to perform complex data manipulations.
Why Choose NumPy Over Python Lists?
NumPy arrays are more efficient and provide better performance for numerical computations compared to native Python lists. The vectorized operations and broadcasting features of NumPy make the code more elegant and readable, enhancing the overall programming experience.
Why is NumPy important in Data Science?
In the field of Data Science, NumPy plays a crucial role due to its ability to efficiently handle and manipulate multidimensional arrays. Its powerful features make it an indispensable tool for various tasks related to data manipulation, machine learning, and scientific computing.
Efficient Data Manipulation:
NumPy arrays, also known as ndarrays, provide a highly efficient way to store and manipulate data. By storing elements of the same type and size, NumPy arrays ensure efficient memory usage and optimized data processing, making them ideal for handling large datasets.
Support for Vectorized Operations:
NumPy offers support for vectorized operations, allowing users to perform element-wise operations on arrays without the need for explicit loops. This feature not only simplifies the code but also improves its performance, making NumPy a preferred choice for numerical computations in Data Science.
Enhanced Data Processing Capabilities:
By leveraging NumPy's array data structure and built-in functions, data scientists can efficiently handle tasks such as finding array dimensions, performing arithmetic operations, and applying statistical functions. This enhances the overall data processing capabilities and productivity of data science projects.
NumPy Basics and Installation
NumPy, short for Numerical Python, is an essential library in Python for working with multidimensional arrays. It provides efficient data structures and high-performance operations, making it ideal for tasks related to data manipulation, machine learning, and scientific computing.
Getting Started with NumPy:
If you are using Anaconda, NumPy comes pre-installed. However, if you need to install NumPy separately, you can do so by running the following command in your terminal:
pip install numpy
After installation, you can import NumPy into your Python code using the following command:
import numpy as np
Creating NumPy Arrays
NumPy arrays, also known as ndarrays, are powerful data structures that allow for efficient handling of multidimensional arrays. These arrays are a fundamental component of NumPy and provide a wide range of functionalities for data manipulation, machine learning, and scientific computing.
Basic NumPy Arrays
To create a basic NumPy array, you can use the np.array()
method. This method takes a list of values as input and creates a NumPy array with those values. For example:
# Basic NumPy Arrays
import numpy as np
# Create a one-dimensional NumPy array with integer values
arr1 = np.array([1, 2, 3, 4])
print(arr1)
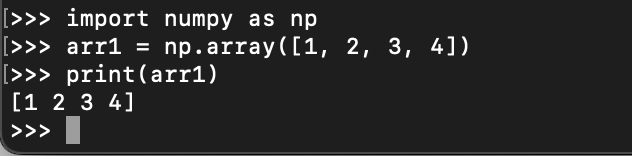
This code snippet creates a one-dimensional NumPy array with integer values.
Specifying Data Types
You can specify the data type of the array elements using the dtype
argument in the np.array()
method. For instance:
# Specifying data type of the array elements
import numpy as np
arr2 = np.array([1, 2, 3, 4], dtype=np.float32)
print(arr2)

This code creates a NumPy array with float values instead of integers.
Multi-dimensional Arrays
NumPy arrays can also be multi-dimensional, allowing you to represent matrices and higher-dimensional data structures. For example:
# Multi-dimensional arrays
import numpy as np
arr3 = np.array([[1, 2, 3], [4, 5, 6]])
print(arr3)

This code snippet creates a two-dimensional NumPy array with two rows and three columns.
Array of Zeros
If you need to create an array filled with zeros, you can use the np.zeros()
method. Simply specify the shape of the desired array:
# Array of Zeros
import numpy as np
arr_zeros = np.zeros((2, 3))
print(arr_zeros)

This code snippet creates a two-dimensional NumPy array filled with zeros, with two rows and three columns.
Indexing and Slicing NumPy Arrays
One of the key features of NumPy arrays is the ability to access individual elements or slices of the array using indexing. NumPy arrays follow zero-based indexing, where the first element is accessed using index 0. For example:
# Indexing and Slicing NumPy Arrays
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
# Accessing individual elements
print(arr[0])
# Slicing
print(arr[1:4])
This code snippet will output the first element of the array, which is 1. You can also slice NumPy arrays to retrieve a subset of elements:
This will output elements from index 1 to 3 (excluding 4) from the array.

Reshaping Arrays
You can reshape NumPy arrays using the reshape()
function, which allows you to change the dimensions of the array without altering the original data. For example:
# Reshaping Arrays
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
new_arr = arr.reshape(1, 5)
print(new_arr)
This will reshape the original 1D array into a 2D array with 2 rows and 3 columns.

Concatenating Arrays
NumPy also provides the concatenate()
function to combine multiple arrays along a specified axis. This is useful for combining data from different sources or performing operations on multiple arrays simultaneously.
# Concatenating Arrays
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
concatenated_arr = np.concatenate((arr1, arr2), axis=1)
print(concatenated_arr)
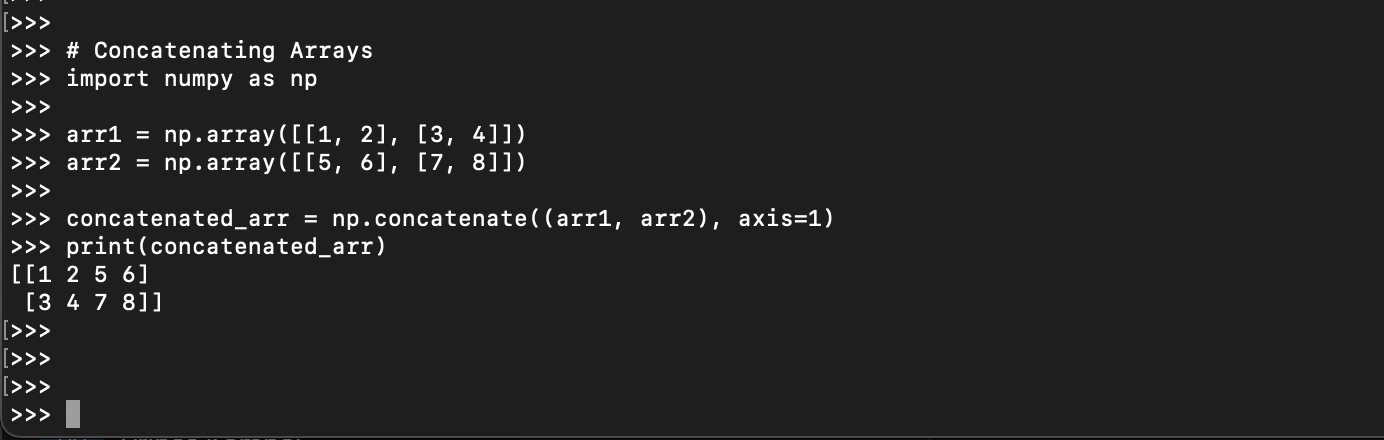
Transposing Arrays
The transpose()
function in NumPy allows you to interchange rows and columns in an array, effectively rotating the array. This operation is commonly used in matrix operations and linear algebra.
# Transposing Arrays
import numpy as np
arr3 = np.array([[1, 2, 3], [4, 5, 6]])
arr_transpose = np.transpose(arr3)
print(arr_transpose)

By mastering the art of accessing and manipulating NumPy arrays, you can leverage the full potential of NumPy for efficient data manipulation, machine learning, and scientific computing tasks.
Multidimensional Arrays in NumPy
NumPy arrays are at the core of the NumPy library, offering powerful capabilities for working with multi-dimensional arrays. These arrays, also known as ndarrays, provide efficient data structures that are essential for tasks related to data manipulation, machine learning, and scientific computing.
Array Indexing and Slicing:
Accessing and manipulating elements within multi-dimensional arrays is made easy with NumPy's indexing and slicing capabilities. This allows for precise selection of data subsets for analysis and processing.
# Array Indexing and Slicing
import numpy as np
# Creating a multi-dimensional array
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Accessing individual elements
print(arr[0, 0]) # Accessing the element at row 0, column 0
# Slicing
print(arr[:, 1]) # Accessing the second column of the array

Matrix Operations:
NumPy's support for multi-dimensional arrays enables efficient matrix operations such as matrix multiplication, transposition, and inversion. These operations are essential for various mathematical computations in data science and machine learning.
# Matrix Operations
import numpy as np
# Matrix multiplication
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
matrix_product = np.dot(matrix1, matrix2)
print(matrix_product)
# Transposition
matrix_transpose = np.transpose(matrix1)
print(matrix_transpose)
# Inversion
matrix_inverse = np.linalg.inv(matrix1)
print(matrix_inverse)
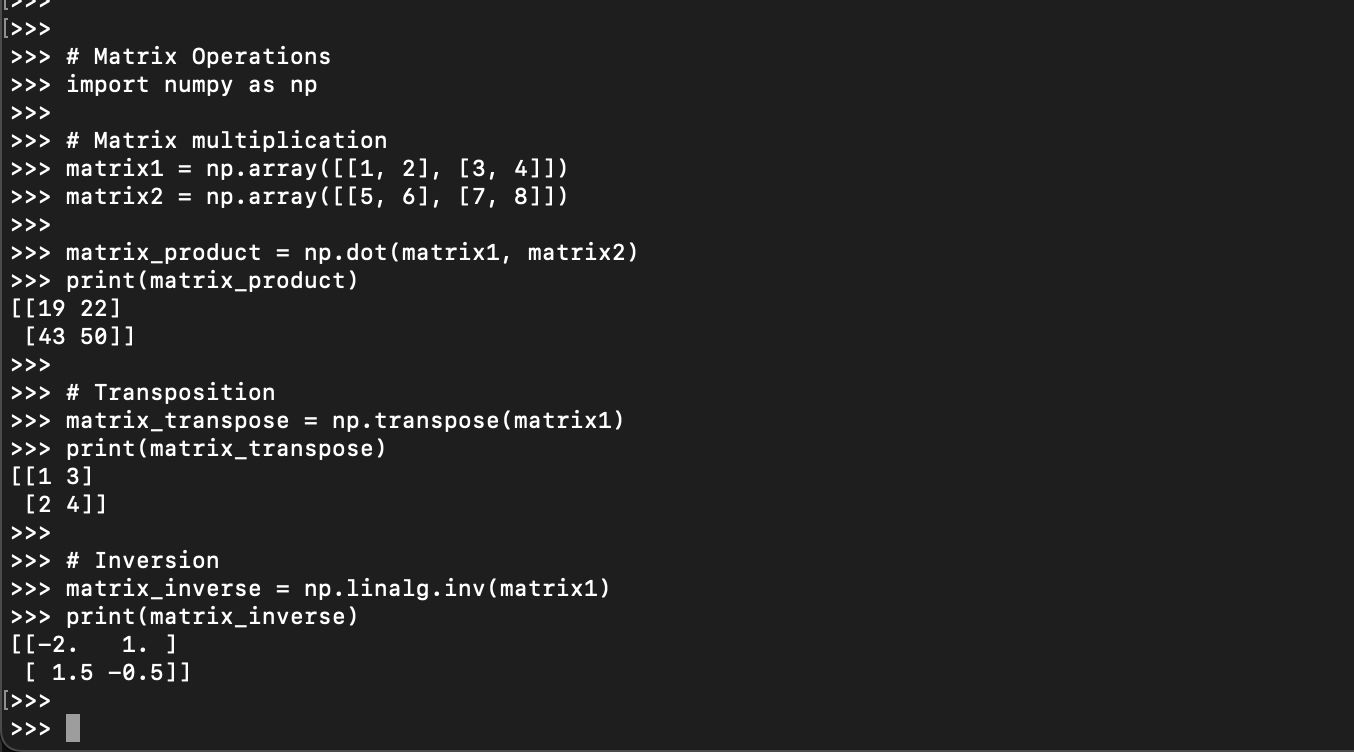
Integration with Machine Learning Libraries:
NumPy arrays seamlessly integrate with popular machine learning libraries like SciPy, Pandas, and Scikit-learn, providing a solid foundation for building and training machine learning models.
NumPy Functions and Vectorized Operations
NumPy, short for Numerical Python, is a powerful library in Python that offers a wide range of functions and capabilities for working with multi-dimensional arrays. These functions, combined with the concept of vectorized operations, make NumPy an essential tool for tasks related to data manipulation, machine learning, and scientific computing.
Vectorized Operations in NumPy:
Vectorized operations in NumPy are at the core of its efficiency and performance benefits. These operations allow for element-wise computations on arrays without the need for explicit loops, making the code more elegant and readable. By leveraging vectorized operations, NumPy ensures faster execution of numerical computations compared to traditional Python lists.
# Vectorized Operations in NumPy
import numpy as np
# Creating NumPy arrays
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([6, 7, 8, 9, 10])
# Element-wise addition
result_addition = arr1 + arr2
print("Element-wise addition:", result_addition)
# Element-wise subtraction
result_subtraction = arr1 - arr2
print("Element-wise subtraction:", result_subtraction)
# Element-wise multiplication
result_multiplication = arr1 * arr2
print("Element-wise multiplication:", result_multiplication)
# Element-wise division
result_division = arr1 / arr2
print("Element-wise division:", result_division)

Advantages of Vectorized Operations:
1. Efficiency: Vectorized operations in NumPy are implemented in compiled C code, making them significantly faster than equivalent Python code.
2. Readability: Vectorized operations simplify the code by eliminating the need for loops, leading to cleaner and more concise code.
3. Performance: By performing operations on entire arrays at once, NumPy's vectorized operations optimize the use of hardware resources and enhance the overall performance of numerical computations.
Overall, NumPy functions and vectorized operations are instrumental in enabling efficient data manipulation, machine learning tasks, and scientific computing endeavors. By mastering these functionalities, users can unlock the full potential of NumPy for their programming and analytical needs.
Data Manipulation with NumPy
NumPy arrays, also known as ndarrays, are powerful data structures that play a crucial role in data manipulation, machine learning, and scientific computing tasks. These arrays offer efficient storage and manipulation of data, making them ideal for handling large datasets and performing numerical computations.
Efficient Data Structures:
NumPy arrays store elements of the same type and size, making them highly efficient for data manipulation tasks such as data science and machine learning. This efficient data structure ensures optimized memory usage and faster processing of data, enhancing the overall performance of numerical computations.
High Performance:
NumPy provides optimized operations on arrays, even as they grow in size, ensuring fast processing and high performance. The vectorized operations and broadcasting features of NumPy make the code more elegant and readable, improving the efficiency of data manipulation tasks.
Python Interface:
NumPy offers a convenient Python interface for working with multi-dimensional arrays, simplifying complex data manipulations. By importing NumPy into your Python code, you can leverage its powerful features to streamline your data processing tasks and enhance your overall programming experience.
Vectorized Operations in NumPy:
Vectorized operations in NumPy are essential for efficient data manipulation and numerical computations. These operations allow for element-wise computations on arrays without the need for explicit loops, improving code readability and execution speed. By leveraging vectorized operations, you can enhance the performance of your data manipulation tasks in NumPy.
Overall, NumPy arrays provide a solid foundation for data manipulation tasks in data science, machine learning, and scientific computing. By understanding and utilizing the features of NumPy arrays, you can optimize your data processing workflows and enhance your programming experience.
Conclusion and Further Learning Resources
In conclusion, NumPy is an indispensable library for beginners and experts alike in the field of data science, machine learning, and scientific computing. Its efficient data structures, high-performance operations, and seamless integration with other libraries make it a fundamental tool for manipulating multidimensional arrays and conducting numerical computations.
FAQ's
- What is NumPy used for?
NumPy is used for numerical computing in Python. It offers extensive support for sizable, multi-dimensional arrays and matrices, accompanied by an array of mathematical functions tailored to efficiently manipulate these arrays. NumPy is widely used in data analysis, scientific computing, machine learning, and more areas where numerical operations are performed extensively. - What is NumPy and Pandas?
NumPy and Pandas are both popular Python libraries used for data manipulation and analysis. NumPy provides support for multi-dimensional arrays and mathematical functions, while Pandas builds upon NumPy and offers additional data structures and functions specifically tailored for data analysis tasks, such as DataFrame and Series. - What is the function of NumPy in Python?
The function of NumPy in Python is to provide support for numerical computing by offering powerful tools for creating, manipulating, and performing operations on arrays and matrices. NumPy simplifies numerical computations by providing efficient implementations of mathematical functions and operations. - What is NumPy with an example?
NumPy is a Python library utilized for numerical computations.. Here's an example of how to create a NumPy array:pythonCopy codeimport numpy as np
arr = np.array([1, 2, 3, 4, 5
])print(arr)
- What is the advantage of NumPy?
The advantages of NumPy include:- Effective handling and manipulation of extensive, multi-dimensional arrays and matrices.
- Fast execution of mathematical operations on arrays without the need for explicit loops.
- Support for a wide range of mathematical functions for array manipulation and computation.
- Integration with other Python libraries for scientific computing and data analysis.
- What is the difference between NumPy and Pandas?
NumPy focuses on providing support for multi-dimensional arrays and mathematical functions, while Pandas provides additional data structures and functions specifically tailored for data analysis tasks, such as DataFrame and Series. NumPy is more suitable for numerical computations, whereas Pandas is more geared towards data manipulation and analysis. - What is the difference between a NumPy array and a list?
NumPy arrays are homogeneous arrays with a fixed size, whereas Python lists can contain elements of different data types and sizes. NumPy arrays offer more efficient storage and operations for numerical computations compared to Python lists. - What are the advantages and disadvantages of NumPy?
Advantages of NumPy:- Efficient storage and manipulation of large arrays and matrices.
- Fast execution of mathematical operations.
- Wide range of mathematical functions for array manipulation.
Disadvantages of NumPy: - Learning curve for beginners.
- Limited support for non-numeric data types compared to Python lists.
- Which is faster, a list or a NumPy array?
NumPy arrays are generally faster than Python lists for numerical computations, especially when dealing with large datasets. This is because NumPy arrays are implemented in C and optimized for performance, whereas Python lists are implemented in Python and involve more overhead. - What is the main function of NumPy in Python?
The main function of NumPy in Python is to provide support for numerical computing by offering efficient tools for creating, manipulating, and performing operations on arrays and matrices. NumPy simplifies numerical computations and enables faster execution of mathematical operations compared to traditional Python data structures.